Navigation Mesh#
Warning
Navigation Mesh is currently in Beta. For more information on pre-release and beta terms, please see the Omniverse License Agreement
Overview#
Navigation Mesh, or “NavMesh”, is a tool used to compute a polygonal mesh that represents the traversable area of one or more agents. From computed polygonal mesh, a linear path between two points can be generated.
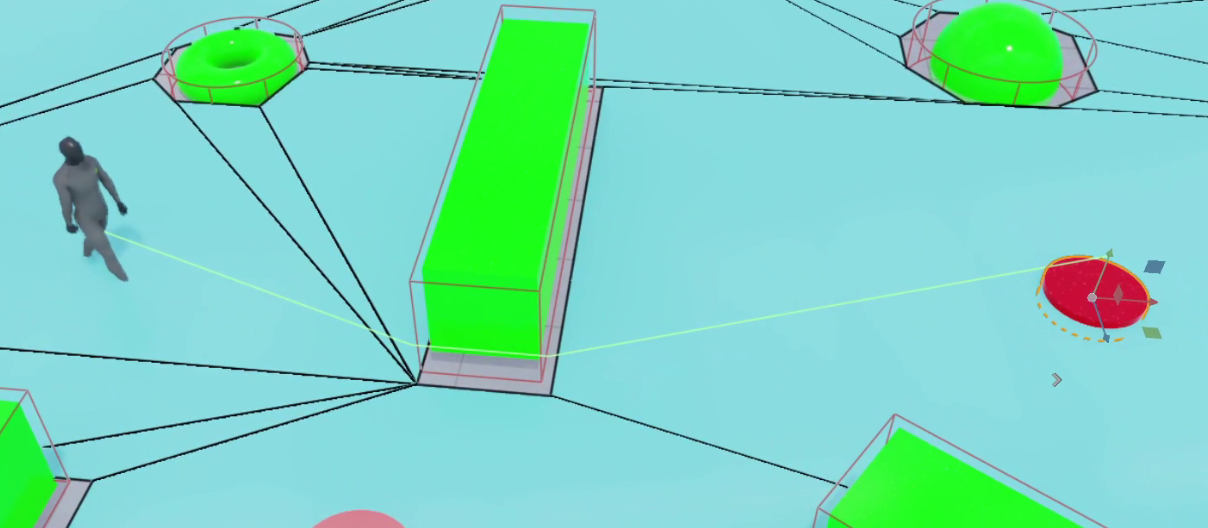