Colliders#
Geometric prims may be used as colliders by applying UsdPhysics.CollisionAPI
. The underlying collision representation is then implicitely created from the USD geometry. If the prim with UsdPhysics.CollisionAPI
or any of its ancestor prims has a UsdPhysics.RigidBodyAPI
, then the collider moves with the dynamic object, otherwise it is considered static.
Note
Collision is not supported for some pairs of colliders depending on their associated rigid body configuration and the simulation mode (CPU or GPU). This is discussed in section Rigid Body Collider Compatibility
Create and Destroy a Static Collider#
The prim created in the following snippet is an example of a static collider. You could swap the cube geometry for a sphere, a mesh, a convex mesh, or any of the supported geometry types outlined in Primitive Geometry Colliders and Mesh Geometry Colliders.
The following Python snippet demonstrates the creation and destruction of a cube collision prim centered at the origin:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
cubePath = "/World/cube"
# Properties of the geometry
cubePosition = Gf.Vec3f(0.0, 0.0, 0.0)
cubeOrientation = Gf.Quatf(1.0)
cubeScale = Gf.Vec3f(1.0, 1.0, 1.0)
cubeSize = 2.0
cubeHalfExtent = cubeSize/2.0
cubeGeom = UsdGeom.Cube.Define(stage, cubePath)
cubeGeom.CreateSizeAttr(cubeSize)
cubeGeom.CreateExtentAttr([Gf.Vec3f(-cubeHalfExtent), Gf.Vec3f(cubeHalfExtent)])
cubeGeom.AddTranslateOp().Set(cubePosition)
cubeGeom.AddOrientOp().Set(cubeOrientation)
cubeGeom.AddScaleOp().Set(cubeScale)
cubePrim = cubeGeom.GetPrim()
UsdPhysics.CollisionAPI.Apply(cubePrim)
stage.RemovePrim(cubePath)
Primitive Geometry Colliders#
Collider functionality can be applied to primitve and mesh geometry types. This section convers primitive types while the following section covers meshes.
The following UsdGeom
types are supported using UsdPhysics.CollisionAPI
. The resulting collision representations precisely map to these geometries:
UsdGeom Type
Example Code Snippets
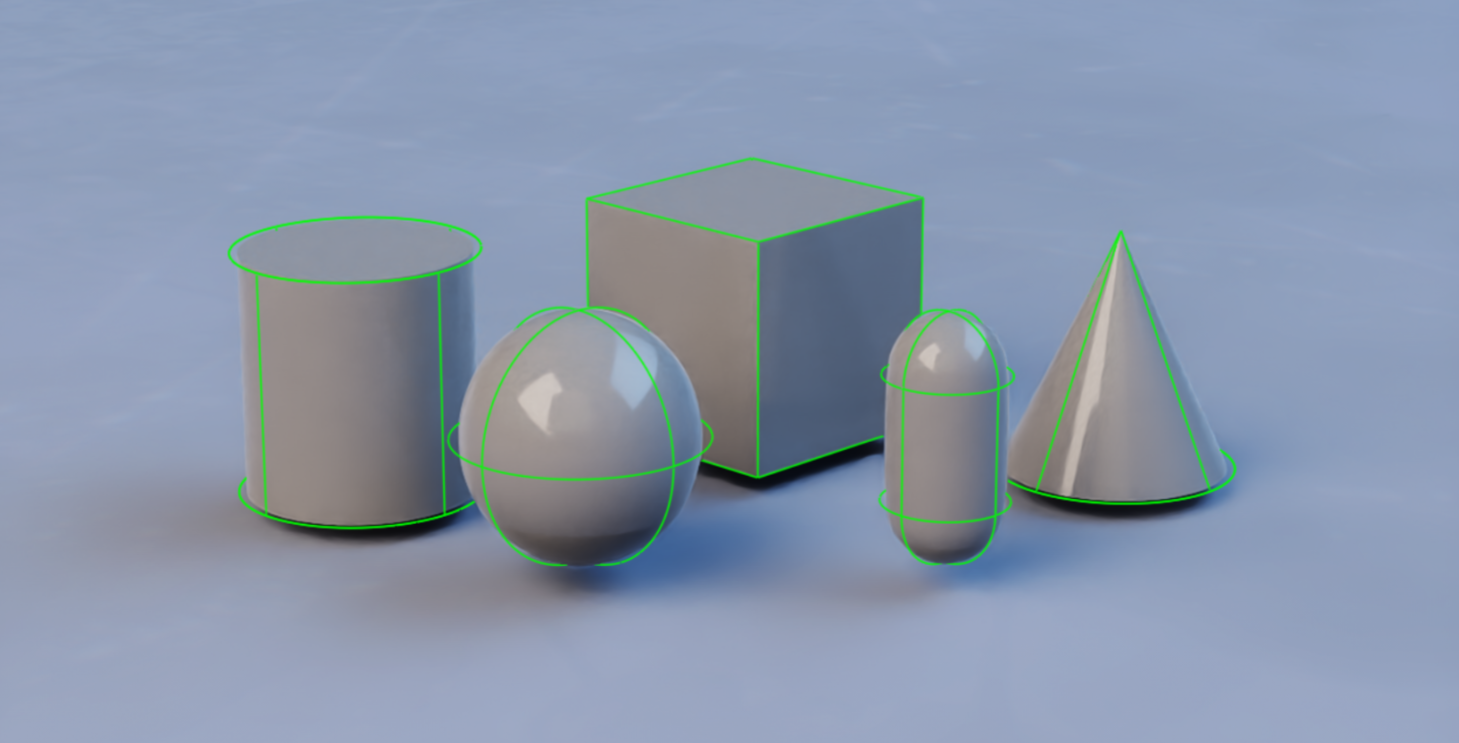
Figure 1 Primitive colliders from left to right: cylinder, sphere, box, capsule, and cone.#
Create a Sphere Collider#
The creation of a sphere collider is similar to the cube example in the preceding section Create and Destroy a Static Collider. The following Python snippet demonstrates how to create a sphere collider:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
spherePath = "/World/sphere"
sphereRadius = 2.0
sphereGeom = UsdGeom.Sphere.Define(stage, spherePath)
sphereGeom.CreateRadiusAttr(sphereRadius)
sphereGeom.AddTranslateOp().Set(Gf.Vec3f(0.0))
sphereGeom.AddOrientOp().Set(Gf.Quatf(1.0))
sphereGeom.AddScaleOp().Set(Gf.Vec3f(1.0))
spherePrim = sphereGeom.GetPrim()
UsdPhysics.CollisionAPI.Apply(spherePrim)
Create a Box Collider#
The creation of a box collider is nearly identical to the cube example in the preceding section Create and Destroy a Static Collider. The only difference here is that a box has non-uniform axis scaling. This is illustrated in the following Python snippet:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
boxPath = "/World/box"
boxDimensions = Gf.Vec3f(2.0, 3.0, 4.0)
boxGeom = UsdGeom.Cube.Define(stage, boxPath)
boxGeom.AddTranslateOp().Set(Gf.Vec3f(0.0))
boxGeom.AddOrientOp().Set(Gf.Quatf(1.0))
boxGeom.AddScaleOp().Set(boxDimensions)
boxPrim = boxGeom.GetPrim()
UsdPhysics.CollisionAPI.Apply(boxPrim)
Create a Capsule Collider#
The creation of a capsule collider is similar to the cube example in the preceding section Create and Destroy a Static Collider. The following Python snippet demonstrates how to create a capsule collider:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
capsulePath = "/World/capsule"
capsuleRadius = 1.0
capsuleHeight = 10.0
capsuleAxis = UsdGeom.Tokens.y
capsuleGeom = UsdGeom.Capsule.Define(stage, capsulePath)
capsuleGeom.AddTranslateOp().Set(Gf.Vec3f(0.0))
capsuleGeom.AddOrientOp().Set(Gf.Quatf(1.0))
capsuleGeom.CreateRadiusAttr(capsuleRadius)
capsuleGeom.CreateHeightAttr(capsuleHeight)
capsuleGeom.CreateAxisAttr(capsuleAxis)
capsulePrim = capsuleGeom.GetPrim()
UsdPhysics.CollisionAPI.Apply(capsulePrim)
Create a Cylinder Collider#
The creation of a cylinder collider is similar to the cube example in the preceding section Create and Destroy a Static Collider. The following Python snippet demonstrates how to create a cylinder collider:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
cylinderPath = "/World/cylinder"
cylinderHeight = 10.0
cylinderRadius = 0.5
cylinderAxis = UsdGeom.Tokens.y
cylinderGeom = UsdGeom.Cylinder.Define(stage, cylinderPath)
cylinderGeom.AddTranslateOp().Set(Gf.Vec3f(0.0))
cylinderGeom.AddOrientOp().Set(Gf.Quatf(1.0))
cylinderGeom.CreateHeightAttr(cylinderHeight)
cylinderGeom.CreateRadiusAttr(cylinderRadius)
cylinderGeom.CreateAxisAttr(cylinderAxis)
cylinderPrim = cylinderGeom.GetPrim()
UsdPhysics.CollisionAPI.Apply(cylinderPrim)
A rounded cylinder may be created by adding the margin attribute to the cylinder geometry prim and setting it to some positive value:
cylinderPrim.CreateAttribute("physxConvexGeometry:margin", Sdf.ValueTypeNames.Float).Set(0.1)
With SETTING_COLLISION_APPROXIMATE_CYLINDERS set to True, all cylinders on the scene will be approximated with convex meshes. Note that approximated cylinders ignore the margin attribute:
from carb.settings import get_settings
from omni.physx.bindings._physx import SETTING_COLLISION_APPROXIMATE_CYLINDERS
...
# Use convex hull approximation
get_settings().set_bool(SETTING_COLLISION_APPROXIMATE_CYLINDERS, True)
Create a Cone Collider#
The creation of a cone collider is similar to the cube example in the preceding section Create and Destroy a Static Collider. The following Python snippet demonstrates how to create a cone collider:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
conePath = "/World/cone"
coneRadius = 1.0
coneHeight = 10.0
coneAxis = UsdGeom.Tokens.y
coneGeom = UsdGeom.Cone.Define(stage, conePath)
coneGeom.AddTranslateOp().Set(Gf.Vec3f(0.0))
coneGeom.AddOrientOp().Set(Gf.Quatf(1.0))
coneGeom.CreateRadiusAttr(coneRadius)
coneGeom.CreateHeightAttr(coneHeight)
coneGeom.CreateAxisAttr(coneAxis)
conePrim = coneGeom.GetPrim()
UsdPhysics.CollisionAPI.Apply(conePrim)
A rounded cone may be created by adding the margin attribute to the cone geometry prim and setting it to some positive value:
conePrim.CreateAttribute("physxConvexGeometry:margin", Sdf.ValueTypeNames.Float).Set(0.1)
With SETTING_COLLISION_APPROXIMATE_CONES set to True, all cones on the scene will be approximated with convex meshes. Note that approximated cones ignore the margin attribute:
from carb.settings import get_settings
from omni.physx.bindings._physx import SETTING_COLLISION_APPROXIMATE_CONES
...
# Use convex hull approximation
get_settings().set_bool(SETTING_COLLISION_APPROXIMATE_CONES, True)
Mesh Geometry Colliders#
Colliders based on UsdGeom.Mesh
with applied UsdPhysics.CollisionAPI
may further be configured using the UsdPhysics.MeshCollisionAPI
:
Mesh Approximation
Implied Collision Geometry
UsdPhysics.Tokens.none
UsdPhysics.Tokens.meshSimplification
UsdPhysics.Tokens.convexHull
UsdPhysics.Tokens.convexDecomposition
UsdPhysics.Tokens.boundingSphere
UsdPhysics.Tokens.boundingCube
PhysxSchema.Tokens.sdf (using
PhysxSchema.PhysxSDFMeshCollisionAPI
)
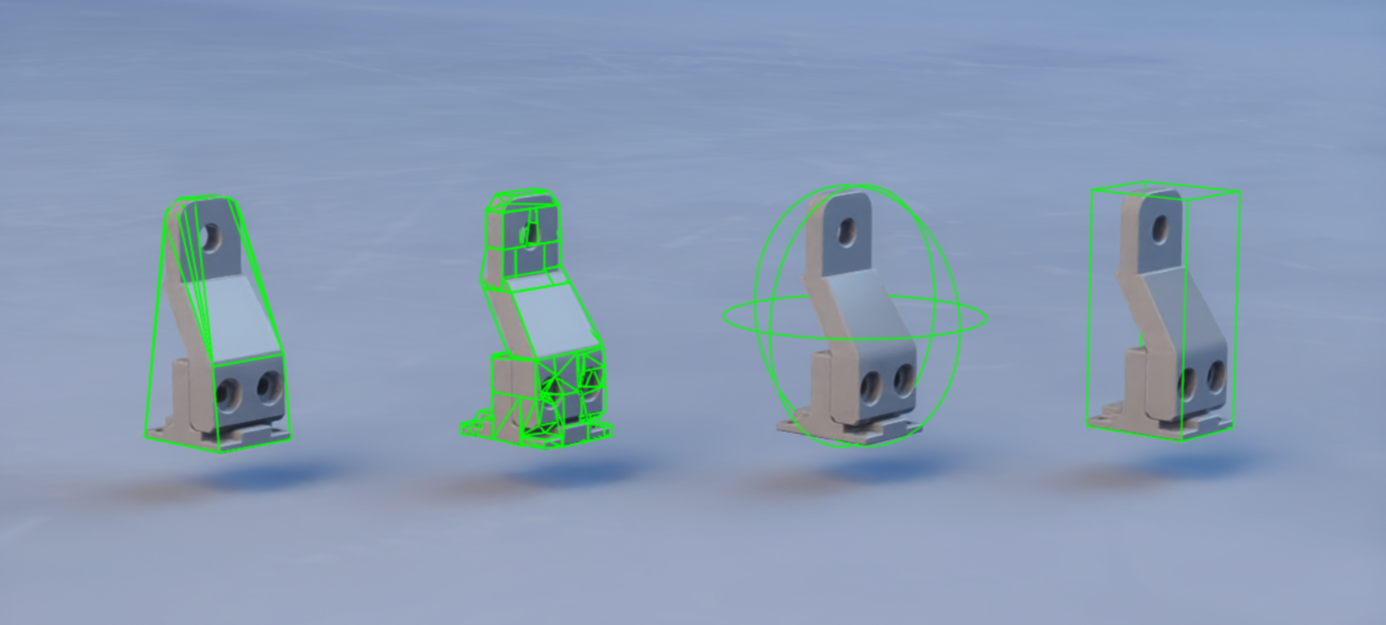
Figure 2 Convex approximation options from left to right: convex hull, convex decomposition, bounding sphere, and bounding cube.#
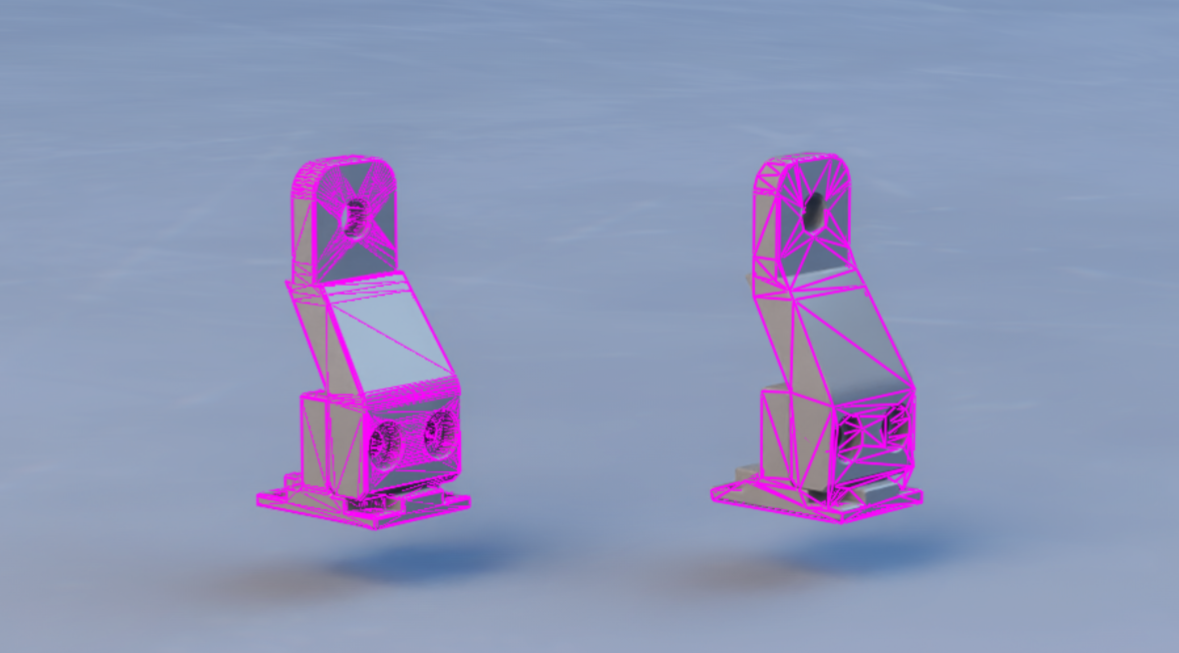
Figure 3 Mesh approximation options: none (full mesh) and with simplification.#
Instead of using a single UsdGeom.Mesh
prim directly, merging multiple source meshes is also supported by using the PhysxSchema.PhysxMeshMergeCollisionAPI
as described here.
Create a Mesh Collider#
All of the approximation types listed above require the application of UsdPhysics.CollisionAPI
and UsdPhysics.MeshCollisionAPI
to a mesh geometry prim. This is illustrated in the following Python snippet:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
halfSize = 0.5
points = [
Gf.Vec3f(halfSize, -halfSize, -halfSize),
Gf.Vec3f(halfSize, halfSize, -halfSize),
Gf.Vec3f(halfSize, halfSize, halfSize),
Gf.Vec3f(halfSize, -halfSize, halfSize),
Gf.Vec3f(-halfSize, -halfSize, -halfSize),
Gf.Vec3f(-halfSize, halfSize, -halfSize),
Gf.Vec3f(-halfSize, halfSize, halfSize),
Gf.Vec3f(-halfSize, -halfSize, halfSize),
]
normals = [
Gf.Vec3f(1, 0, 0),
Gf.Vec3f(1, 0, 0),
Gf.Vec3f(1, 0, 0),
Gf.Vec3f(1, 0, 0),
Gf.Vec3f(-1, 0, 0),
Gf.Vec3f(-1, 0, 0),
Gf.Vec3f(-1, 0, 0),
Gf.Vec3f(-1, 0, 0),
]
indices = [
0, 1, 2, 3,
1, 5, 6, 2,
3, 2, 6, 7,
0, 3, 7, 4,
1, 0, 4, 5,
5, 4, 7, 6
]
vertexCounts = [4, 4, 4, 4, 4, 4]
meshOptions = [
UsdPhysics.Tokens.none,
UsdPhysics.Tokens.meshSimplification,
UsdPhysics.Tokens.convexHull,
UsdPhysics.Tokens.convexDecomposition,
UsdPhysics.Tokens.boundingSphere,
UsdPhysics.Tokens.boundingCube
]
for i in range(6):
meshPath = "/World/mesh" + str(i)
mesh = UsdGeom.Mesh.Define(stage, meshPath)
mesh.CreateFaceVertexCountsAttr(vertexCounts)
mesh.CreateFaceVertexIndicesAttr(indices)
mesh.CreatePointsAttr(points)
mesh.CreateDoubleSidedAttr(False)
mesh.CreateNormalsAttr(normals)
meshPrim = mesh.GetPrim()
collisionAPI = UsdPhysics.CollisionAPI.Apply(meshPrim)
meshCollisionAPI = UsdPhysics.MeshCollisionAPI.Apply(meshPrim)
meshCollisionAPI.GetApproximationAttr().Set(meshOptions[i])
For the purpose of simplicity, the Python snippet above creates a mesh that represents a cube. The mesh is then configured to each desired collision type after applying UsdPhysics.MeshCollisionAPI
.
Create a Mesh Merging Collider#
In complex scenes, PhysxSchema.PhysxMeshMergeCollisionAPI
can be used with UsdPhysics.CollisionAPI
to create mesh colliders composed of multiple meshes.
PhysxSchema.PhysxMeshMergeCollisionAPI
, applied to a UsdGeom.Xformable
prim, provides a collection that defines the meshes belonging to the collider. When the implicit collision geometry is created, the given approximation is computed, not based on individual meshes, but on a mesh that is merged from all the meshes enumerated by the collection.
The meshes that get merged may be sourced from anywhere in the stage hierarchy.
Note
If any of the meshes listed in the collection are descendants of a rigid body prim, then they will move together with the rigid body. Any remaining meshes listed in the collection will not move with the rigid body. An example might be a hierarchy with meshes C and D being the children of rigid body prim B and B being the child of mesh prim A. If A, C and D are all added to the merged mesh collection then only C and D will move with rigid body B. This is a natural consequence of the UsdPhysics specification, which allows only rigid body transforms to be updated.
Python example snippet:
from pxr import Usd, UsdGeom, UsdPhysics, Gf, PhysxSchema
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
rigidBodyXform = UsdGeom.Xform.Define(stage, "/World/rigidBody")
UsdPhysics.RigidBodyAPI.Apply(rigidBodyXform.GetPrim())
# Apply also the collision definitions here
UsdPhysics.CollisionAPI.Apply(rigidBodyXform.GetPrim())
collisionMeshAPI = UsdPhysics.MeshCollisionAPI.Apply(rigidBodyXform.GetPrim())
collisionMeshAPI.GetApproximationAttr().Set(UsdPhysics.Tokens.convexHull)
# Define shared mesh points
halfSize = 0.5
points = [
Gf.Vec3f(halfSize, -halfSize, -halfSize),
Gf.Vec3f(halfSize, halfSize, -halfSize),
Gf.Vec3f(halfSize, halfSize, halfSize),
Gf.Vec3f(halfSize, -halfSize, halfSize),
Gf.Vec3f(-halfSize, -halfSize, -halfSize),
Gf.Vec3f(-halfSize, halfSize, -halfSize),
Gf.Vec3f(-halfSize, halfSize, halfSize),
Gf.Vec3f(-halfSize, -halfSize, halfSize),
]
normals = [
Gf.Vec3f(1, 0, 0),
Gf.Vec3f(1, 0, 0),
Gf.Vec3f(1, 0, 0),
Gf.Vec3f(1, 0, 0),
Gf.Vec3f(-1, 0, 0),
Gf.Vec3f(-1, 0, 0),
Gf.Vec3f(-1, 0, 0),
Gf.Vec3f(-1, 0, 0),
]
indices = [
0, 1, 2, 3,
1, 5, 6, 2,
3, 2, 6, 7,
0, 3, 7, 4,
1, 0, 4, 5,
5, 4, 7, 6
]
vertexCounts = [4, 4, 4, 4, 4, 4]
for i in range(3):
meshPath = "/World/rigidBody/mesh" + str(i)
mesh = UsdGeom.Mesh.Define(stage, meshPath)
mesh.CreateFaceVertexCountsAttr(vertexCounts)
mesh.CreateFaceVertexIndicesAttr(indices)
mesh.CreatePointsAttr(points)
mesh.CreateDoubleSidedAttr(False)
mesh.CreateNormalsAttr(normals)
if i == 1:
mesh.AddTranslateOp().Set(Gf.Vec3f(1.0 * i, 0.0, 1.0))
else:
mesh.AddTranslateOp().Set(Gf.Vec3f(1.0 * i, 0.0, 0.0))
# Now setup the mesh merging to include mesh 0 and mesh 2, excluding mesh 1
meshMergeCollision = PhysxSchema.PhysxMeshMergeCollisionAPI.Apply(
rigidBodyXform.GetPrim()
)
meshMergeCollection = meshMergeCollision.GetCollisionMeshesCollectionAPI()
meshMergeCollection.GetIncludesRel().AddTarget("/World/rigidBody")
meshMergeCollection.GetExcludesRel().AddTarget("/World/rigidBody/mesh1")
Create an SDF Collider#
The Signed-Distance-Field (SDF) collision detection feature allows the simulation of dynamic and kinematic rigid bodies with high-detail mesh based colliders. Demos like Nuts and Bolts and Chair Stacking leverage the SDF feature to simulate the contact-rich interaction between dynamic objects with highly non-convex shapes. SDFs can help to speed up collision detection for any mesh based colliders and are a requirement for dynamic rigid bodies with non-convex colliders without resorting to convex decomposition (see section Rigid Body Collider Compatibility).
Note
Multi-material triangle-mesh colliders are not supported with the SDF collision feature.
For general and SDF-specific advice on tuning colliders, check Improve Collision Behavior.
For more details, see the paper Factory: Fast Contact for Robotic Assembly
This code snippet shows how to enable SDF collision on a mesh geometry prim:
sdfMeshPrim = ...
# Apply CollisionAPI
UsdPhysics.CollisionAPI.Apply(sdfMeshPrim)
# Apply MeshCollisionAPI and set approximation to SDF
meshCollision = UsdPhysics.MeshCollisionAPI.Apply(sdfMeshPrim)
meshCollision.CreateApproximationAttr(PhysxSchema.Tokens.sdf)
# Apply PhysxSDFMeshCollisionAPI and set resolution
sdfMeshCollision = PhysxSchema.PhysxSDFMeshCollisionAPI.Apply(sdfMeshPrim)
sdfMeshCollision.CreateSdfResolutionAttr(300)
For high-throughput access to SDF samples and gradients, use the SdfShapeView
provided by the Omni Physics Tensors, which supports on-GPU access.
Sparse SDFs#
The SDF is computed on a uniform grid with a grid spacing equal to the mesh’s longest AABB extent divided by an SDF Resolution attribute. In addition to dense SDFs, PhysX supports sparse SDFs featuring a hierarchical structure with a reduced density of samples in regions that are far from any surface. This provides considerable memory savings at similar contact fidelity. Sparse SDFs are enabled when PhysxSDFMeshCollisionAPI.sdfSubgridResolution
is nonzero.
Sparse SDFs consist of a background SDF with coarsely spaced samples and high-resolution subgrids. These are blocks of finely spaced samples in areas close to the surface. A subgrid is created in the cube formed by 8 neighboring background SDF samples whenever this space contains points with an absolute distance value below the narrowBandThickness. If the coarse SDF is already accurate to a conservative error threshold, e.g. in areas with planar geometry, no subgrid is created. In the figure below, the subgrid resolution is 5.
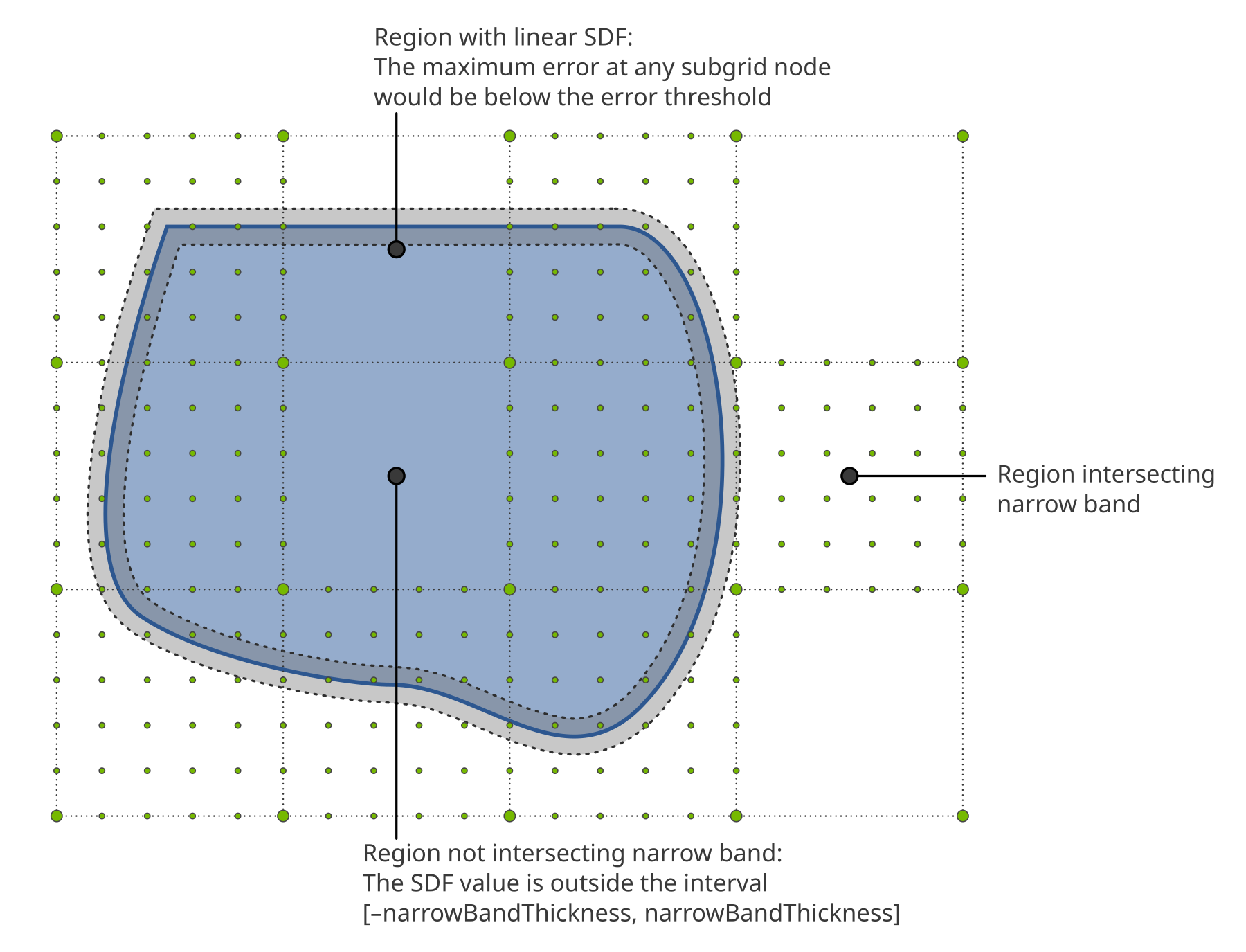
A sparse SDF consists of a background SDF and subgrids in the vicinty of surfaces.
Generate Mesh Colliders (Cooking)#
The process that generates collision approximations from mesh data is commonly referred to as cooking. The resulting output of the cooking operation is referred to as cooking data. A completely transparent caching system has been added to avoid recomputing such cooking data over and over again.
Cooking behaviour can be customized changing one of the following settings:
SETTING_USE_LOCAL_MESH_CACHE: Enables or disables the cooking data cache. Disabling it means recomputing coolider data from scratch every time it will be needed.
SETTING_SAVE_COOKED_DATA: Enables or disables saving cooked data to USD (legacy, defaults to disabled). Enabling it will increase the data of USD file.
SETTING_LOCAL_MESH_CACHE_SIZE_MB: Defines the maximum disk size of the local mesh cache. This is just an hint to the caching system.
SETTING_UJITSO_COLLISION_COOKING: Uses the newer UJITSO system to handle caching. Disabling it can be useful to compare behaviour with the legacy cooking system.
SETTING_UJITSO_COOKING_MAX_PROCESS_COUNT: Changes the maximum number of UJITSO processes dedicated to cooking computation.
Note
Options are not persistent and they must be set to the wanted value at every boot
To change the settings using code use the carb.settings interfaces, for example:
import carb
import omni.physx.bindings._physx as physx_bindings
# Acquire settings interface
settings = carb.settings.get_settings()
# Check existing values
save_cooked_data = settings.get_as_bool(physx_bindings.SETTING_SAVE_COOKED_DATA)
use_local_cache = settings.get_as_bool(physx_bindings.SETTING_USE_LOCAL_MESH_CACHE)
# Disable saving cooked data to USD and disable cooking cache
settings.set_bool(physx_bindings.SETTING_SAVE_COOKED_DATA, False)
settings.set_bool(physx_bindings.SETTING_USE_LOCAL_MESH_CACHE, False)
Cooking data for all simulated objects must be available before starting a simulation in order to create the required internal data structures used for collision detection. For this reason, when starting a Simulation the main thread will be blocked until all cooked data is read from the cache. If cooking data is not available from the cache, it will be computed, contributing to further blocking of the main thread.
Configure Collision Filtering#
This section describes two methods to disable collision between different colliders.
Collision Group Filtering#
Collision group filtering allows collision detection between pairs of colliders to be disabled (or enabled), based on collision groups. Every collider in a collision group collides with every other in that group. More importantly, collision groups may be configured with a list of other collision groups such that the accumulation of colliders in that list do not collide with the colliders in the group that owns the list. By default, the lists are opt-out; that is, the default behavior is that every group collides with every other and the lists determine pairs of groups that do not collide. It is possible to invert this behavior so that the lists are opt-in; that is, the lists specify the group pairs that do collide and the default behavior is no pairs collide.
The following snippet implements a basic example of collision filtering using UsdPhysics.CollisionGroup
:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# The goal is to create three colliders (box0, box1, box2) with collision
# only between box0 and box1.
# This is achieved by creating three collision groups such that each group
# contains a different box.
# Filtering is used to filter out (or in) collision between collision groups.
# The process is repeated without inverted collision filtering (opt-out) and with
# inverted collision group filtering (opt-in)
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
useInvertedCollisionGroups = [False, True]
for k in range(2):
# Three collision groups. Each group has a list of members and
# a list of other collision groups used to filter collision.
collisionGroupPaths = [
"/World/collisionGroup0",
"/World/collisionGroup1",
"/World/collisionGroup2"]
collisionGroupIncludesRel = [None] * 3
collisionGroupFilteredRels = [None] * 3
# Three boxes. Each box will be added to its corresponding collision group.
boxPaths = ["/World/boxA", "/World/boxB", "/World/boxC"]
# Set the flag that configures inverted collision group filtering as required.
if useInvertedCollisionGroups[k]:
defaultPrimPath = str(stage.GetDefaultPrim().GetPath())
physicsScenePath = defaultPrimPath + "/physicsScene"
physicsScene = UsdPhysics.Scene.Define(stage, physicsScenePath)
physicsScenePrim = physicsScene.GetPrim()
physxSceneAPI = PhysxSchema.PhysxSceneAPI.Apply(physicsScenePrim)
physxSceneAPI.CreateInvertCollisionGroupFilterAttr(True)
# Create three colliders
for i in range(3):
boxGeom = UsdGeom.Cube.Define(stage, boxPaths[i])
boxPrim = boxGeom.GetPrim()
UsdPhysics.RigidBodyAPI.Apply(boxPrim)
UsdPhysics.CollisionAPI.Apply(boxPrim)
# Create three collision groups.
# For each collision group create a list that will store the colliders in the group
# For each collision group create a list of other collision groups
for i in range(3):
collisionGroup = UsdPhysics.CollisionGroup.Define(stage, collisionGroupPaths[i])
collisionGroupPrim = collisionGroup.GetPrim()
collectionAPI = Usd.CollectionAPI.Apply(
collisionGroupPrim,
UsdPhysics.Tokens.colliders
)
collisionGroupIncludesRel[i] = collectionAPI.CreateIncludesRel()
collisionGroupFilteredRels[i] = collisionGroup.CreateFilteredGroupsRel()
# Add box0 to collisionGroup0, box1 to collisonGroup1, box2 to collisionGroup2
collisionGroupIncludesRel[0].AddTarget(boxPaths[0])
collisionGroupIncludesRel[1].AddTarget(boxPaths[1])
collisionGroupIncludesRel[2].AddTarget(boxPaths[2])
if useInvertedCollisionGroups[k]:
# Collision only between box0 and box1 is achieved by fil tering in
# collision between collisionGroup0 and collisionGroup2
collisionGroupFilteredRels[0].AddTarget(collisionGroupPaths[1])
else:
# Collision only between box0 and box1 is achieved by filtering out
# collision between collisionGroup0 and collisionGroup2 and between
# collisionGroup1 and collisionGroup2
collisionGroupFilteredRels[0].AddTarget(collisionGroupPaths[2])
collisionGroupFilteredRels[1].AddTarget(collisionGroupPaths[2])
Pairwise Collision Filtering#
In cases where using collision groups is insufficiently fine-grained, it is possible to disable collision between specific stage hierarchy pairs using UsdPhysics.FilteredPairsAPI
. Pairwise filtering takes precedence over collision group filtering.
The provided filteredPairs
relationship defines which hierarchies should be filtered with the given pair. Note that the filtering does not have an exclude relationship. As a consequence, all child objects (colliders, rigid bodies, articulations) will be filtered.
The following Python snippet illustrates the use of UsdPhysics.FilteredPairsAPI
:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
# The goal is to create three colliders (box0, box1, box2) with
# collision only between box0 and box1.
# This is achieved by disabling collision between box0 and box2 and
# between box1 and box2
# Create three collider prims
boxPaths = ["/World/boxA", "/World/boxB", "/World/boxC"]
boxPrims = [None] * 3
for i in range(3):
boxGeom = UsdGeom.Cube.Define(stage, boxPaths[i])
boxPrim = boxGeom.GetPrim()
UsdPhysics.RigidBodyAPI.Apply(boxPrim)
UsdPhysics.CollisionAPI.Apply(boxPrim)
boxPrims[i] = boxPrim
# Disable collision between box0 and box2
filteredPairsAPIBox0 = UsdPhysics.FilteredPairsAPI.Apply(boxPrims[0])
filteredPairsAPIBox0.CreateFilteredPairsRel().AddTarget(boxPaths[2])
# Disable collision between box1 and box2
filteredPairsAPIBox1 = UsdPhysics.FilteredPairsAPI.Apply(boxPrims[1])
filteredPairsAPIBox1.CreateFilteredPairsRel().AddTarget(boxPaths[2])
Configure Rest and Contact Offsets#
The attributes rest offset and collision offset allow contact detection and response to be tuned to resolve a variety of problems associated with finite simulation timestep and discrepancies between collision and render geometry. Both of these are attributes governed by applying PhysxSchema.PhysxCollisionAPI
to a collider prim.
The Collision Offset defines a distance from the surface of the collision geometry at which contacts start being generated. The default value will result in an auto-generated value that takes account of scene gravity, simulation timestep, and geometry extent. It is recommended to increase this parameter, if you have fast-moving or thin objects that tunnel through each other over a single simulation timestep. It is important to note that increasing the collision offset may result in a performance penalty because it can result in the generation of more contacts. An alternative is to enable continuous collision detection (CCD) on the rigid body, as discussed in section Configure Continuous Collision Detection on a Rigid Body.
The Rest Offset defines a small distance from the surface of the collision geometry at which the effective contact with the shape takes place. It can be positive, zero or negative, and may be useful in cases where the visualization mesh is for example slightly smaller than the collision geometry. Setting an appropriate negative rest offset results in the contact occurring at the visually correct distance.
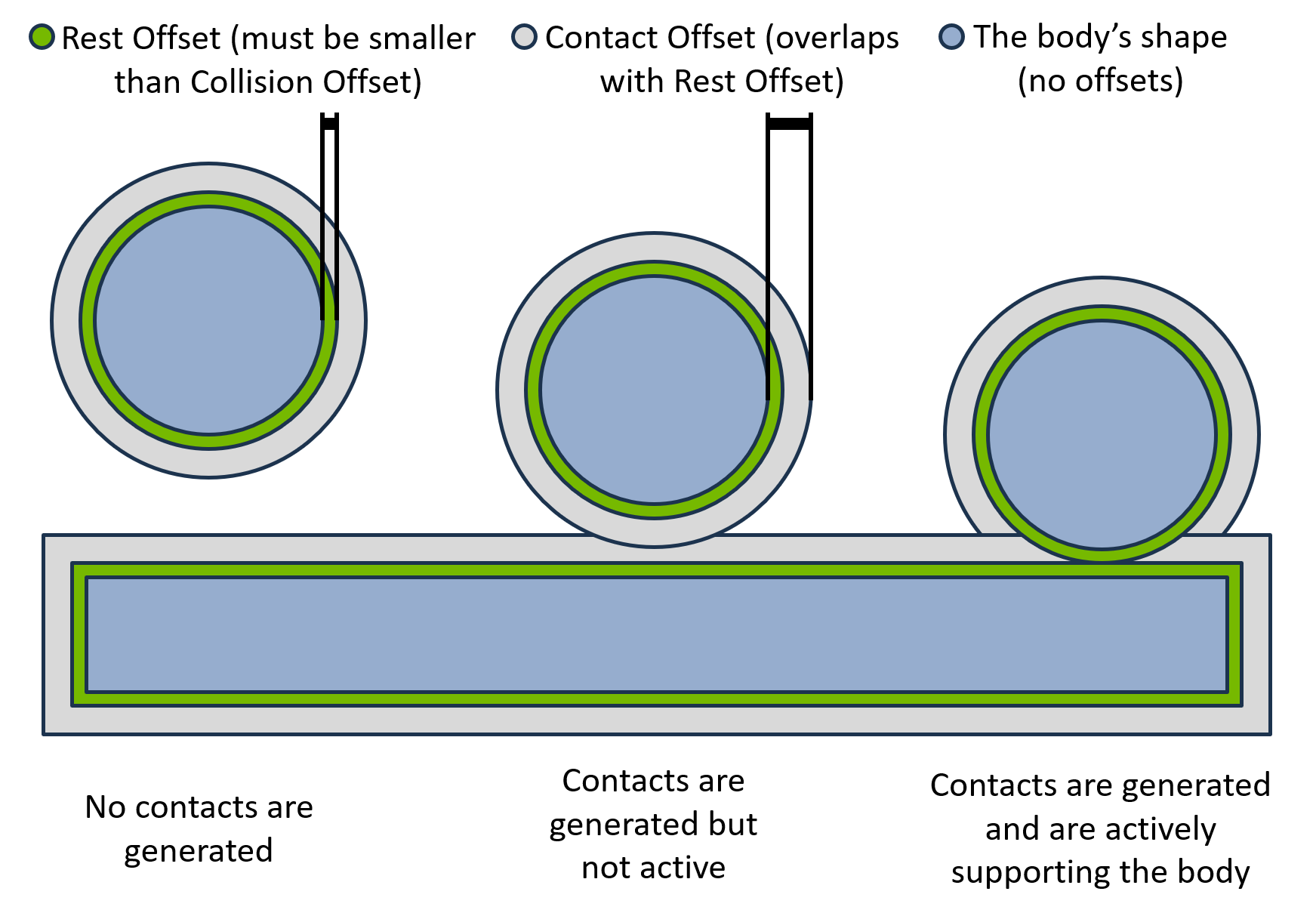
Figure 4 The effects of rest and collision offsets.#
Contact Reports can be used if information about contacts needs to be known at runtime, see Contact Reports.
The following Python snippet illustrates the application of PhysxSchema.PhysxCollisionAPI
:
from pxr import Usd, UsdGeom, UsdPhysics, Gf
import omni.usd
# Create a stage
omni.usd.get_context().new_stage()
stage = omni.usd.get_context().get_stage()
# Define the root Xform (transformable object)
rootxform = UsdGeom.Xform.Define(stage, "/World")
cubePath = "/World/cube"
# rest and collision offset
restOffset = 0.0
contactOffset = 0.02
# Properties of the geometry
cubePosition = Gf.Vec3f(2.0, 0.0, 0.0)
cubeOrientation = Gf.Quatf(1.0)
cubeScale = Gf.Vec3f(1.0, 1.0, 1.0)
cubeSize = 2.0
cubeHalfExtent = cubeSize/2.0
# Create a cube geometry prim
cubeGeom = UsdGeom.Cube.Define(stage, cubePath)
cubeGeom.CreateSizeAttr(cubeSize)
cubeGeom.CreateExtentAttr([Gf.Vec3f(-cubeHalfExtent), Gf.Vec3f(cubeHalfExtent)])
cubeGeom.AddTranslateOp().Set(cubePosition)
cubeGeom.AddOrientOp().Set(cubeOrientation)
cubeGeom.AddScaleOp().Set(cubeScale)
cubePrim = cubeGeom.GetPrim()
UsdPhysics.CollisionAPI.Apply(cubePrim)
# Apply PhysxSchema.PhysxCollisionAPI to the cube prim
physxCollisionAPI = PhysxSchema.PhysxCollisionAPI.Apply(cubePrim)
physxCollisionAPI.CreateRestOffsetAttr(restOffset)
physxCollisionAPI.CreateContactOffsetAttr(contactOffset)