3.4. OmniGraph: Python Scripting
While OmniGraph is intended to be a visual scripting tool, it does have python scripting interfaces. This tutorial will give a simple example of how to script an action graph using Python.
3.4.1. Getting Started
Prerequisites
Review the GUI Tutorial series, especially OmniGraph and Interactive Scripting prior to beginning this tutorial.
Review the Core API Tutorial series, especially Hello World to become familiar with the extension workflow via Python.
Run the OmniGraph Keyboard Example following Keyboard Inputs.
The layout of the Action Graph is seen in the image below.
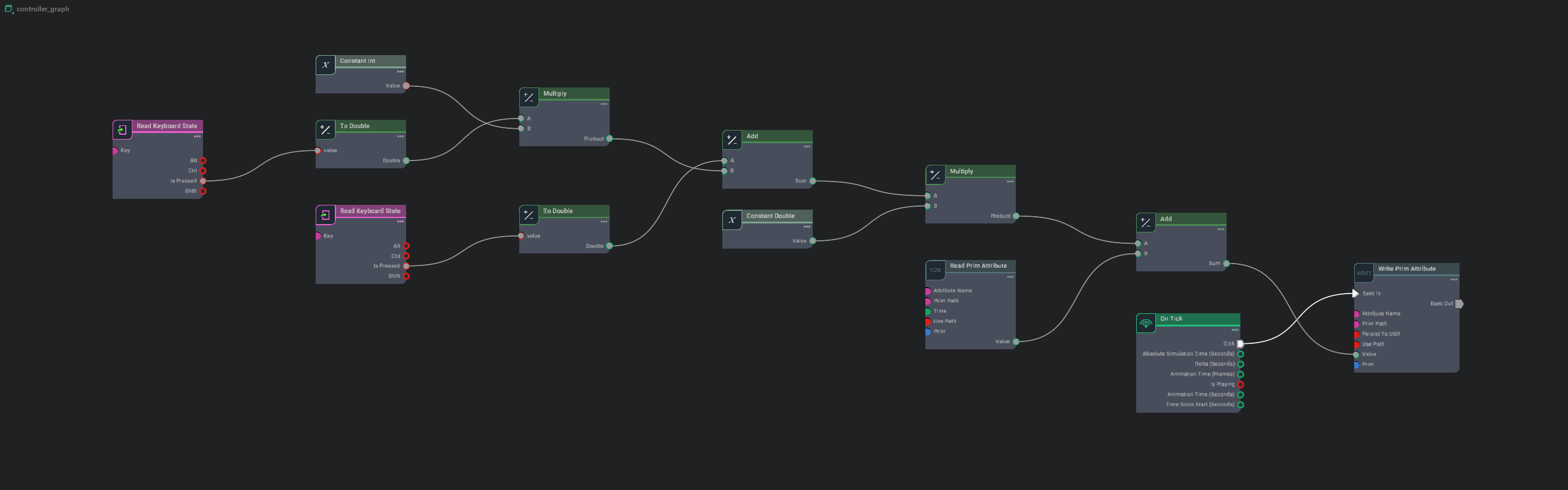
While you can make the graph above using the GUI exclusively, you can also compose the same graph via Python.
3.4.2. Code Explained
Make sure the OmniGraph Keyboard example panel is open. Click the Open Containing Folder button on the upper right corner of the panel to open the folder that contains the source code.
Open the file
omnigraph_keyboard.py
, find thesetup_scene()
section.
Inside the scene setup, we first add a cube and a ground plane to the scene, and fix the camera view.
1 world = self.get_world()
2 world.scene.add(
3 VisualCuboid(
4 prim_path="/Cube", # The prim path of the cube in the USD stage
5 name="cube", # The unique name used to retrieve the object from the scene later on
6 position=np.array([0, 0, 10.0]), # Using the current stage units which is cms by default.
7 scale=np.array([10.0, 10, 10]), # most arguments accept mainly numpy arrays.
8 color=np.array([0, 1.0, 1.0]), # RGB channels, going from 0-1
9 )
10 )
11 world.scene.add_default_ground_plane()
12 set_camera_view(eye=np.array([75, 75, 45]), target=np.array([0, 0, 0]))
To compose the graph, the steps are: Get handle to the graph editor, create the nodes, set the values of any nodes, and then make the connections between the nodes explicitly.
1 # setup graph
2 keys = og.Controller.Keys
3 og.Controller.edit(
4 {"graph_path": "/controller_graph", "evaluator_name": "execution"},
5 {
6 keys.CREATE_NODES: [
7 ("OnTick", "omni.graph.action.OnTick"),
8 ("A", "omni.graph.nodes.ReadKeyboardState"),
9 ("D", "omni.graph.nodes.ReadKeyboardState"),
10 ("ToDouble1", "omni.graph.nodes.ToDouble"),
11 ("ToDouble2", "omni.graph.nodes.ToDouble"),
12 ("Negate", "omni.graph.nodes.Multiply"),
13 ("DeltaAdd", "omni.graph.nodes.Add"),
14 ("SizeAdd", "omni.graph.nodes.Add"),
15 ("NegOne", "omni.graph.nodes.ConstantInt"),
16 ("CubeWrite", "omni.graph.nodes.WritePrimAttribute"), # write prim property translate
17 ("CubeRead", "omni.graph.nodes.ReadPrimAttribute"),
18 ],
19 keys.SET_VALUES: [
20 ("A.inputs:key", "A"),
21 ("D.inputs:key", "D"),
22 ("OnTick.inputs:onlyPlayback", True), # only tick when simulator is playing
23 ("NegOne.inputs:value", -1),
24 ("CubeWrite.inputs:name", "size"),
25 ("CubeWrite.inputs:primPath", "/Cube"),
26 ("CubeWrite.inputs:usePath", True),
27 ("CubeRead.inputs:name", "size"),
28 ("CubeRead.inputs:primPath", "/Cube"),
29 ("CubeRead.inputs:usePath", True),
30 ],
31 keys.CONNECT: [
32 ("OnTick.outputs:tick", "CubeWrite.inputs:execIn"),
33 ("A.outputs:isPressed", "ToDouble1.inputs:value"),
34 ("D.outputs:isPressed", "ToDouble2.inputs:value"),
35 ("ToDouble2.outputs:converted", "Negate.inputs:a"),
36 ("NegOne.inputs:value", "Negate.inputs:b"),
37 ("ToDouble1.outputs:converted", "DeltaAdd.inputs:a"),
38 ("Negate.outputs:product", "DeltaAdd.inputs:b"),
39 ("DeltaAdd.outputs:sum", "SizeAdd.inputs:a"),
40 ("CubeRead.outputs:value", "SizeAdd.inputs:b"),
41 ("SizeAdd.outputs:sum", "CubeWrite.inputs:value"),
42 ],
43 },
44 )
Note
For input that are bundle types, such as the “Prim” input inside the WritePrimAttribute node, you may not be able to set their values this way. This is circumvented by allowing users to specify a path or a token to the bundle target. In those cases, make sure the “Use Path” option box is checked.
3.4.3. Summary
In this tutorial, we introduced scripting OmniGraph via Python.
3.4.3.1. Further Reading
For more Python Scripting in OmniGraph