Distribute Tool
Overview
The Distribute Tool is used to distribute selected prims along any axis. Prims can be distributed between two endpoints or within a specific bounding box area. A variety of options exist to modify how the selected prims are distributed for the most desired results.
Set Up
Enable the Extension
Using the Distribute Tool begins with enabling the extension.
Check if it’s Enabled
You can quickly see if the extension is already loaded by looking at the menu bar. If your menu bar contains the Tools
menu with Distribute
appearing as a menu item, then the Distribute Tool is loaded.
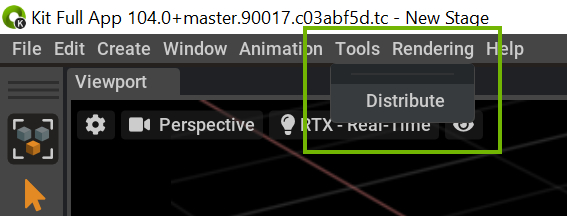
How to Enable
To enable the extension:
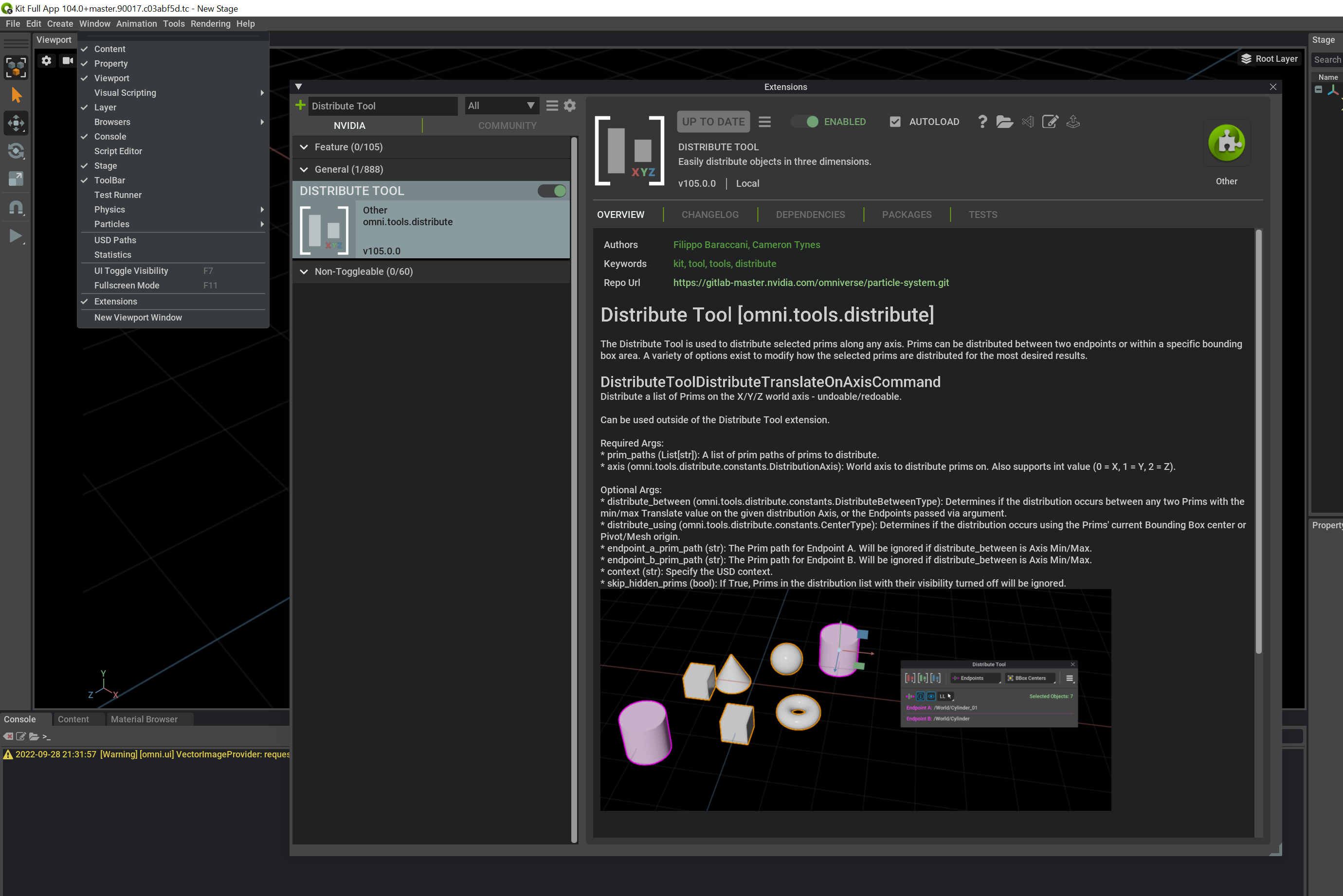
Navigate to
window > extensions
In the search bar enter
Distribute Tool
Locate the Distribute Tool extension and select it.
Select the
Enabled
toggle to enable the extension.Select the
Check Mark
next to auto-load to Load the Extension Automatically on App Start if desired.Close the Extensions Panel.
At this point the extension should be enabled and you should now see the tool menu item appear in your menu bar.
User Guide
Open the Distribute Tool
To open the Distribute Tool, simply select Distribute
from the Tools
menu. A checkmark will appear when the tool is open.
Clicking on Distribute
again will close the Distribute Tool.
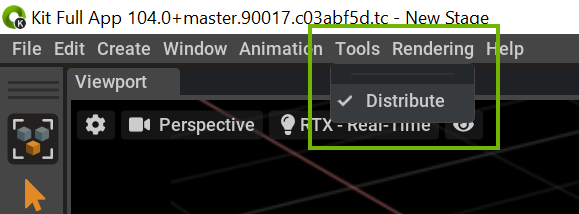
Features
The standard features of the Distribute Tool.
Distribution Axes
The axes buttons are clicked on to apply a distribution operation to a current valid selection. Distribution is supported on all 3 axes: X, Y, and Z.
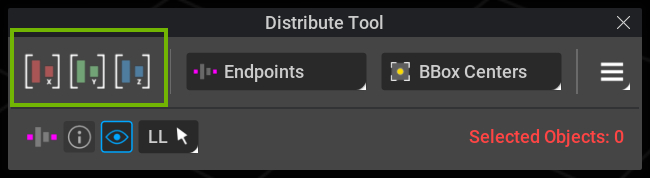
Feature |
Description |
---|---|
X |
Click to distribute along the X axis.
|
Y |
Click to distribute along the Y axis.
|
Z |
Click to distribute along the Z axis.
|
Note
Distribution is applied in world space. If a parent and child are selected while running a distribute operation, their world positions will be handled individually.
Distribute Between
The distribute between drop-down menu allows users to choose how they would like to distribute the selected prims, ie. which bounds to distribute between.
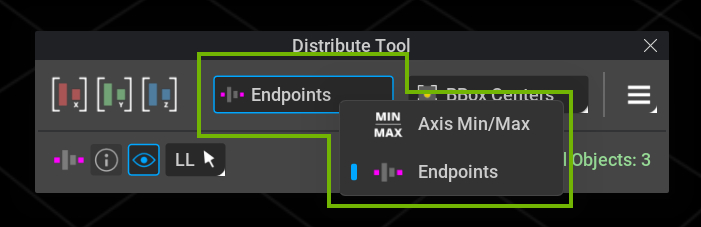
Feature |
Description |
---|---|
Axis Min/Max |
Determines the objects with the min/max translation values on the given distribution axis and distributes the remaining objects between them.
|
Endpoints |
Uses the selected endpoints and distributes the remaining objects between them.
|
Distribute Using
The distribute using drop-down menu allows users to choose how selected prims will be processed for distribution spacing calculations.
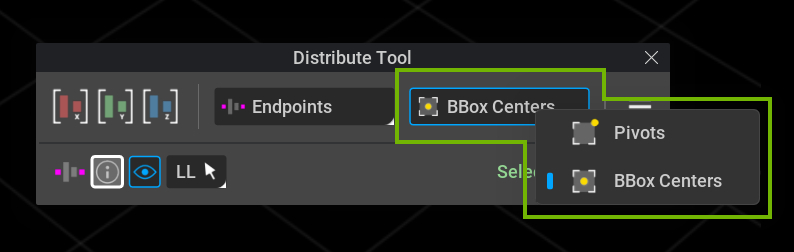
Feature |
Description |
---|---|
Pivots |
Uses the mesh origin or pivot (if available) of the selected objects to determine the spacing of the distribution.
|
BBox Centers |
Uses the centroid of the selected objects to determine the spacing of the distribution. This method uses the combined Centroid if multiple boundable objects are nested.
|
Endpoint Options
The endpoint options provides additional functionality when the Distribute Between
method is set to Endpoints
, such as choosing between different endpoint selection methods and toggling graphical overlays.
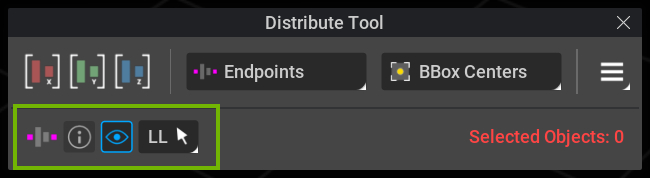
Endpoints Info
When enabled, the selected endpoints’ paths will be displayed in the tool UI.
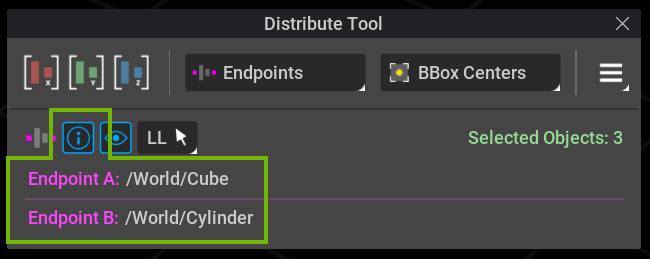
Endpoints Viewport Overlay
When enabled, the endpoints will be displayed with a pink overlay in the viewport.
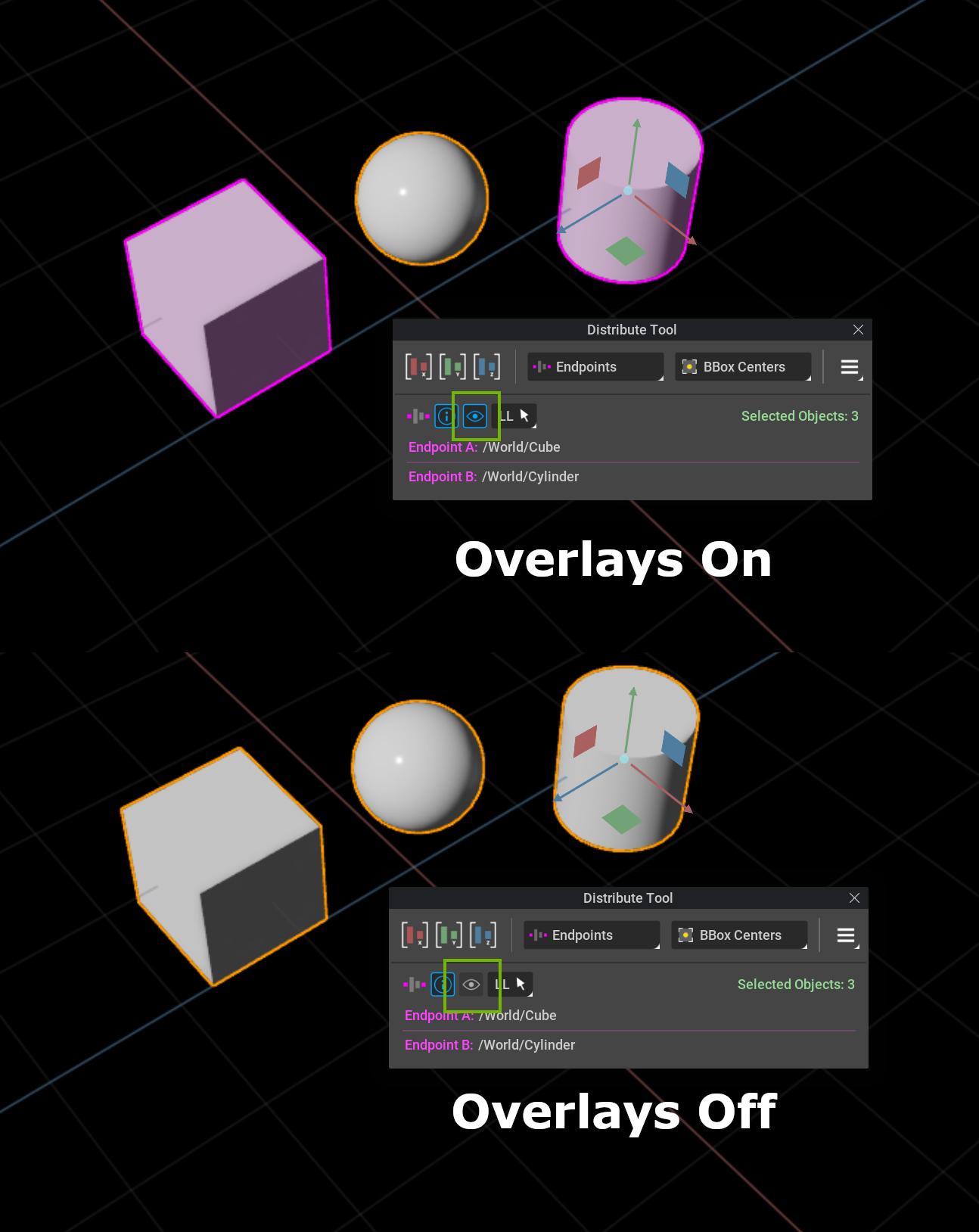
Endpoints Selection Method
This drop-down allows the user to choose how the endpoint prims are decided based on selection order.
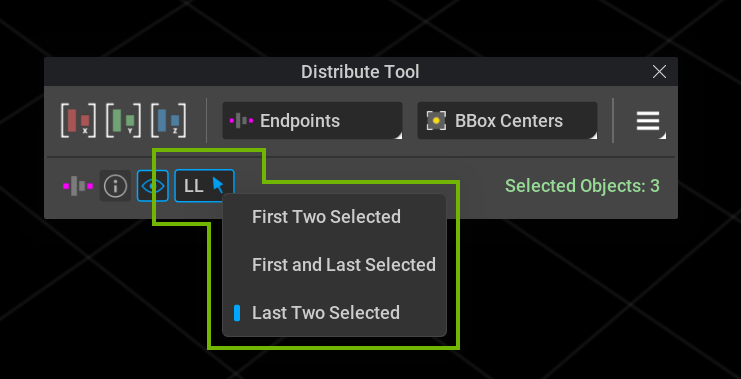
Feature |
Description |
---|---|
FF |
First Two Selected. The first two objects selected will be used as the endpoints for distribution.
|
FL |
First and Last Selected. The first and last objects selected will be used as the endpoints for distribution.
|
LL |
Last Two Selected. The last two objects selected will be used as the endpoints for distribution.
|
Programming Guide
The Distribute Tool can also be used without the UI via Omniverse Commands. This way, other scripts and extensions can use the core Distribute logic to distribute a list of provided prims along an axis.
The DistributeToolDistributeTranslateOnAxisCommand
is used to distribute a list of prims via command. It expects the following arguments:
Argument |
Description |
---|---|
prim_paths (list) |
The list of prim paths to distribute.
|
axis (omni.tools.distribute.constants.DistributionAxis) |
The world axis to distribute the prims on. Also supports int values (0 = X, 1 = Y, 2 = Z).
|
Optional Argument |
Description |
---|---|
distribute_between (omni.tools.distribute.constants.DistributeBetweenType) |
Determines if distribution occurs between any two prims with the min/max translate value on the given distribution axis, or the endpoints passed via argument.
|
distribute_using (omni.tools.distribute.constants.CenterType) |
Determines if the distribution occurs using the prims’ current Bounding Box center or Pivot/Mesh origin.
|
endpoint_a_prim_path (str) |
The prim path for Endpoint A. Will be ignored if distribute_between is set to Axis Min/Max.
|
endpoint_b_prim_path (str) |
The prim path for Endpoint B. Will be ignored if distribute_between is set to Axis Min/Max.
|
context (str) |
Specify the USD context. Allows this command to be used in multiple USD contexts.
|
skip_hidden_prims (bool) |
If True, prims in the distribution list with their visibility turned off will be ignored.
|
Below is an example code block where we call the DistributeToolDistributeTranslateOnAxisCommand
and pass in the expected values and prims.
"""
This is a simple example that uses omni.tools.distribute to distribute selected prims on the X axis between two endpoints.
"""
import omni.kit
import omni.usd
from omni.tools.distribute import constants as d_c
usd_context = omni.usd.get_context()
selection = usd_context.get_selection()
selected_prim_paths = selection.get_selected_prim_paths() # Get the selected prim paths
endpoint_a = "/World/Cube" # Set Endpoint A
endpoint_b = "/World/Cube_01" # Set Endpoint B
axis = d_c.DistributionAxis.X # Set the distribution axis to the X axis
distribute_between = d_c.DistributeBetweenType.ENDPOINTS # Distribute between two endpoints
distribute_using = d_c.CenterType.BBOX_CENTER # Use BBox Centers for spacing
omni.kit.commands.execute(
"DistributeToolDistributeTranslateOnAxisCommand",
prim_paths=selected_prim_paths,
axis=axis,
distribute_between=distribute_between,
distribute_using=distribute_using,
endpoint_a_prim_path=endpoint_a,
endpoint_b_prim_path=endpoint_b,
)
In this example we will pass an int
into the axis
field instead of the expected enum, change the distribute_between
value to axis min/max
, and use pivots
for the distribute_using
value.
import omni.kit
import omni.usd
from omni.tools.distribute import constants as d_c
usd_context = omni.usd.get_context()
selection = usd_context.get_selection()
selected_prim_paths = selection.get_selected_prim_paths() # Get the selected prim paths
axis = 2 # Set the distribution axis to the Z axis using an int
distribute_between = d_c.DistributeBetweenType.AXIS_MIN_MAX # Distribute between the axis min/max
distribute_using = d_c.CenterType.PIVOTS # Use Pivots for spacing
omni.kit.commands.execute(
"DistributeToolDistributeTranslateOnAxisCommand",
prim_paths=selected_prim_paths,
axis=axis,
distribute_between=distribute_between,
distribute_using=distribute_using,
)
Features Supported
Works on all USD Xformable types, this includes xforms, meshes, lights, cameras, skeletons, etc.
Works on all prim types, including instanceable, referenced, and payloads.
Supports nested prims
Supports objects with pivots and non-zero pivots.
Supports undo and redo of operations, both from the UI and via command.
Limitations
Objects with time sampled transform ops are ignored and will not produce results when using the Distribute Tool UI or command.
Distribute Tool UI values can not be keyframed for animation.