Develop a Project
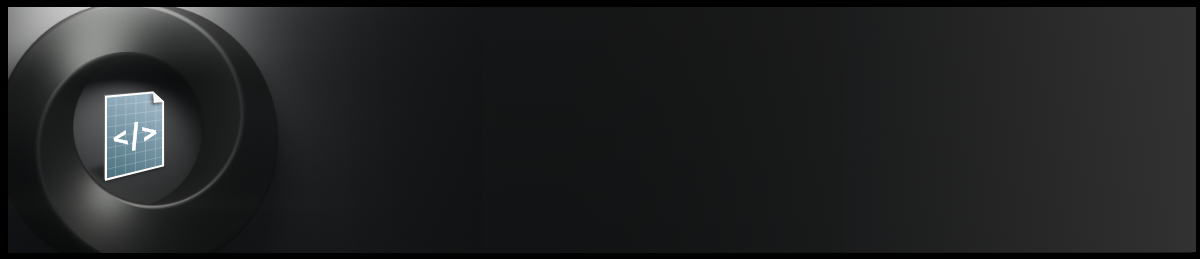
After creating a new Project, the development phase begins. In this phase, you configure and use an assortment of tools and extensions, along with automated documentation features to fit the needs of your project.
Having followed the methods outlined in the Create section, you’ve produced configuration files and established a folder setup. Now you will transform this set of default files to enable new functionality. This stage of Omniverse Project Development is undeniably the most in-depth, offering numerous paths to achieve desired outcomes as a developer.
In this section, we’ll discuss tools and resources for project development, be it crafting an Extension (or multiple extensions), Application, Service, or Connector.
Configure TOML Files
Both Omniverse Applications and Extensions fundamentally rely on a configuration file in TOML format. This file dictates dependencies and settings that the Kit SDK loads and executes. Through this mechanism, Applications can include Extensions, which may further depend on other Extensions, forming a dependency tree.
For details on constructing this tree and the corresponding settings for each Extension, it’s essential to understand the specific configuration files. Applications utilize the .kit file, while Extensions are defined using .toml files. For more on each type of configuration file, please refer to the tabs above.
Extensions can contain many types of assets, such as images, python files, data files, C++ code/header files, documentation, and more. However, one thing all Extensions have in common is the extension.toml file.
Extension.toml should be located in the ./config
folder of your project so that it can be found by various script tools.
Here is an example extension.toml file that can be found in the Advanced Template Repository:
[package]
version = "1.0.0"
title = "Simple UI Extension Template"
description = "The simplest python extension example. Use it as a starting point for your extensions."
# One of categories for UI.
category = "Example"
# Keywords for the extension
keywords = ["kit", "example"]
# Path (relative to the root) or content of readme markdown file for UI.
readme = "docs/README.md"
# Path (relative to the root) of changelog
changelog = "docs/CHANGELOG.md"
# URL of the extension source repository.
repository = "https://github.com/NVIDIA-Omniverse/kit-project-template"
# Icon to show in the extension manager
icon = "data/icon.png"
# Preview to show in the extension manager
preview_image = "data/preview.png"
# Use omni.ui to build simple UI
[dependencies]
"omni.kit.uiapp" = {}
# Main python module this extension provides, it will be publicly available as "import my.hello.world".
[[python.module]]
name = "my.hello.world"
Here we will break this down…
[package]
version = "1.0.0"
This sets the version of your extension. It is critical that this version is set any time you produce a new release of your extension, as this version is most often used to differentiate releases of extensions in registries and databases. As a best practice, it is useful to maintain semantic versioning.
It is also best practice to ensure that you document changes you have made to your code. See the Documentation section for more information.
title = "Simple UI Extension Template"
description = "The simplest python extension example. Use it as a starting point for your extensions."
category = "Example"
keywords = ["kit", "example"]
The title
and description
can be used in registries and publishing destinations to allow users more information on what your extension is used for.
The category
sets an overall filter for where this extension should appear in various UIs.
The keywords
property lists an array of searchable, filterable attributes for this extension.
[dependencies]
"omni.kit.uiapp" = {}
This section is critical to the development of all aspects of your project. The dependencies section in your toml files specifies which extensions are required. As a best practice, you should ensure that you use the smallest list of dependencies that still accomplishes your goals. When setting dependencies for extensions, ensure you only add extensions that are dependencies of that extension.
The brackets {}
in the dependency line allow for parameters such as the following:
order=[ordernum]
allows you to define by signed integer which order the dependencies are loaded. Lower integers are loaded first. (e.g.order=5000
)version=["version ID"]
lets you specify which version of an extension is loaded. (e.g.version="1.0.1"
)exact=true
(default is false) - If set to true, parser will use only an exact match for the version, not just a partial match.
[[python.module]]
name = "my.hello.world"
This section should contain one or more named python modules that are used by the extension. The name is expected to also match a folder structure within the extension path. In this example, the extension named my.hello.world
would have the following path.
my/hello/world
.
These are the minimum required settings for extensions and apps. We will discuss more settings later in the Dev Guide, and you can find plenty of examples of these configuration files in the Developer Reference sections of the menu.
Applications are not much different than extensions. It is assumed that an application is the “root” of a dependency tree. It also often has settings in it related to the behavior of a particular workflow. Regardless, an App has the same TOML file configuration as extensions, but an App’s TOML file is called a .kit file.
.kit files should be located in the ./source/apps
folder of your project so that it can be found by various script tools.
Here is an example kit file that provides some of the minimum settings you’ll need. Additional settings and options can be found later:
[package]
version = "1.0.0"
title = "My Minimum App"
description = "A very simple app."
# One of categories for UI.
category = "Example"
# Keywords for the extension
keywords = ["kit", "example"]
# Path (relative to the root) or content of readme markdown file for UI.
readme = "docs/README.md"
# Path (relative to the root) of changelog
changelog = "docs/CHANGELOG.md"
# URL of the extension source repository.
repository = "https://github.com/NVIDIA-Omniverse/kit-project-template"
# Icon to show in the extension manager
icon = "data/icon.png"
# Preview to show in the extension manager
preview_image = "data/preview.png"
# Use omni.ui to build simple UI
[dependencies]
"omni.kit.uiapp" = {}
Here we will break this down…
[package]
version = "1.0.0"
This sets the version of your extension or app. It is critical that this version is set any time you produce a new release of your extension, as this version is most often used to differentiate releases of extensions/apps in registries and databases. As a best practice, it is useful to maintain semantic versioning https://semver.org/.
It is also best practice to ensure that you document changes you have made in your docs show each version you’ve released.
title = "Simple UI Extension Template"
description = "A very simple app."
category = "Example"
keywords = ["kit", "example"]
The title
and description
can be used in registries and publishing destinations to allow users more information on what your app or extension is used for.
The category
sets an overall filter for where this project should appear in various UIs.
The keywords
property lists an array of searchable, filterable attributes for this extension.
[dependencies]
"omni.kit.uiapp" = {}
This section is critical to the development of all aspects of your project. The dependencies section in your toml files specifies which extensions are to be used by the app. As a best practice, you should ensure that you use the smallest list of dependencies that still accomplishes your goals. And, in extensions especially, you only add dependencies which THAT extension requires.
The brackets {}
in the dependency line allow for parameters such as the following:
order=[ordernum]
allows you to define by signed integer which order the dependencies are loaded. Lower integers are loaded first. (e.g.order=5000
)version=["version ID"]
lets you specify which version of an extension is loaded. (e.g.version="1.0.1"
)exact=true
(default is false) - If set and true, parser will use only an exact match for the version, not just a partial match.
These are the minimum required settings for Apps. We will discuss more settings later in the Dev Guide, and you can find plenty of examples of these configuration files in the Developer Reference sections of the menu.
Available Extensions
Virtually all user-facing elements in an Omniverse Application, such as Omniverse USD Composer or Omniverse USD Presenter, are created using Extensions. The very same extensions used in Omniverse Applications are also available to you for your own development.
The number of extensions provided by both the Community and NVIDIA is continually growing to support new features and use cases. However, a core set of extensions is provided alongside the Omniverse Kit SDK. These ensure basic functionality for your Extensions and Applications including:
Omniverse UI Framework : A UI toolkit for creating beautiful and flexible graphical user interfaces within extensions.
Omni Kit Actions Core : A framework for creating, registering, and discovering programmable Actions in Omniverse.
Omni Scene UI : Provides tooling to create great-looking 3d manipulators and 3d helpers with as little code as possible.
And more.
A list of available Extensions can be found via API Search.
Documentation
If you are developing your project using Repo Tools, you also have the ability to create documentation from source files to be included in your build. This powerful feature helps automate html-based documentation from human-readable .md files.
By running
repo docs
you will generate in the _build/docs/[project_name]/latest/ folder a set of files which represents the html version of your source documentation. The “home page” for your documentation will be the index.html file in that folder.
You can find latest information by reading the Omniverse Documentation System.
Note
You may find that when running repo docs
you receive an error message instead of the build proceeding. If this is the case it is likely that you are either using a project that does not contain the “docs” tool OR that your repo.toml file is not setup correctly. Please refer to the repo tools documentation linked to above for more information.