Extension: omni.kit.window.filepicker-2.10.34 |
Documentation Generated: Jul 17, 2024 |
Overview
The file picker extension provides a standardized dialog for picking files. It is a wrapper around the FileBrowserWidget
,
but with reasonable defaults for common settings, so it’s a higher-level entry point to that interface.
Nevertheless, users will still have the ability to customize some parts but we’ve boiled them down to just the essential ones.
Why you should use this extension:
Checkpoints fully supported if available on the server.
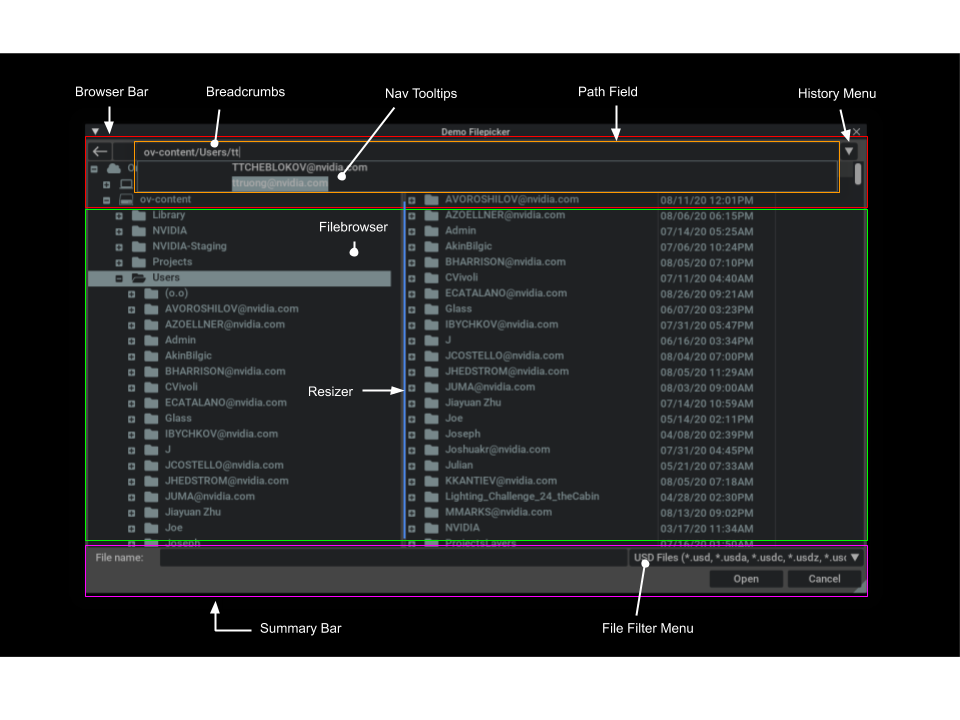
Quickstart
You can pop-up a dialog in just 2 steps. First, create a dialog.
dialog = FilePickerDialog("Demo Filepicker")
Then, invoke its show_window method.
dialog.show()
Customizing the Dialog
You can customize these parts of the dialog.
Title - The title of the dialog.
Collections - Which of these collections, [“bookmarks”, “omniverse”, “my-computer”] to display.
Filename Url - Url of the file to process.
Postfix options - Show only files of these content types.
Extension options - Show only files with these filename extensions.
Apply label - Label for the apply button.
Apply handler - User provided callback to handle the apply process.
Note that these settings are applied when you show the window. Therefore, each time it’s displayed, the dialog can be tailored to the use case.
Filter files by type
The user has the option to filter what files get shown in the list view.
def on_filter_item(dialog: FilePickerDialog, item: FileBrowserItem) -> bool:
if not item or item.is_folder:
return True
if dialog.current_filter_option == 0:
# Show only files with listed extensions
_, ext = os.path.splitext(item.path)
if ext in [".usd", ".usda", ".usdc", ".usdz"]:
return True
else:
return False
else:
# Show All Files (*)
return True
Options
A common need is to provide user options for the file picker.
def options_pane_build_fn(selected_items):
with ui.CollapsableFrame("Reference Options"):
with ui.HStack(height=0, spacing=2):
ui.Label("Prim Path", width=0)
return True
On apply handler
Provide a handler for when the ok button is clicked.
def on_click_open(dialog: FilePickerDialog, filename: str, dirname: str):
"""
The meat of the App is done in this callback when the user clicks 'Accept'. This is
a potentially costly operation so we implement it as an async operation. The inputs
are the filename and directory name. Together they form the fullpath to the selected
file.
"""
# Normally, you'd want to hide the dialog
# dialog.hide()
dirname = dirname.strip()
if dirname and not dirname.endswith("/"):
dirname += "/"
fullpath = f"{dirname}{filename}"
print(f"Opened file '{fullpath}'.")
Demo app
A complete demo, that includes the code snippets above, is included with this extension at Python.